本文实现了经典蓝牙的查找、配对、连接,低功耗蓝牙的查找、连接、通信。源码见 github 项目 AndroidBluetooth。
1.Android系统蓝牙
1.1 Android系统支持经典蓝牙和低功耗蓝牙:
- Classic Bluetooth(经典蓝牙)。
用于大量的通信时,例如:音频、短信和电话。
- Bluetooth Low Energy(低功耗蓝牙)。
用于低频的通信时,例如:心率监测器、无线键盘,要求Android4.3及更高版本。
1.2 蓝牙堆栈的常规结构
蓝牙应用通过 Binder 与蓝牙进程进行通信。蓝牙进程使用 JNI 与蓝牙堆栈通信,并向开发者提供对各种蓝牙配置文件的访问权限。如下图:
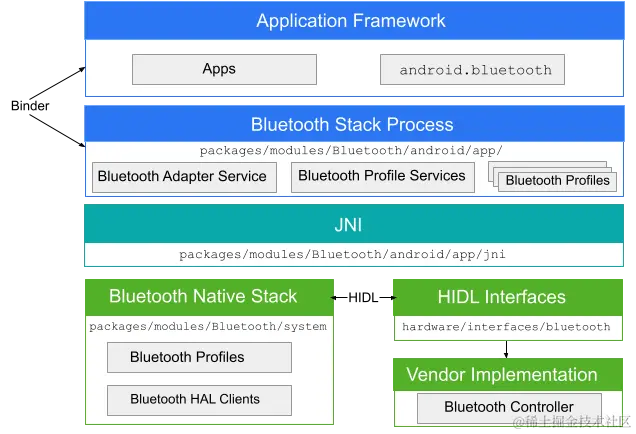
2.经典蓝牙
2.1 申请蓝牙权限
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <!--蓝牙 API30及以下需要申请4个权限,API31及以上时权限列表将不会列出这些权限--> <!--蓝牙 连接、通信--> <uses-permission android:name="android.permission.BLUETOOTH" android:maxSdkVersion="30" /> <!--蓝牙 扫描、设置--> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN" android:maxSdkVersion="30" /> <!--蓝牙 API30及以下需要定位权限 --> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" android:maxSdkVersion="30" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" android:maxSdkVersion="30" />
<!--蓝牙 API31及以上需要申请3个权限--> <!--蓝牙 扫描,可不申请定位权限--> <uses-permission android:name="android.permission.BLUETOOTH_SCAN" android:usesPermissionFlags="neverForLocation" tools:targetApi="s" /> <!--蓝牙 广播,被发现--> <!--<uses-permission android:name="android.permission.BLUETOOTH_ADVERTISE" />--> <!--蓝牙 连接、通信--> <uses-permission android:name="android.permission.BLUETOOTH_CONNECT" />
|
2.2 设置蓝牙
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| BluetoothManager bluetoothManager = context.getSystemService(BluetoothManager.class); BluetoothAdapter bluetoothAdapter = bluetoothManager.getAdapter();
IntentFilter intentFilter = new IntentFilter(); intentFilter.addAction(BluetoothDevice.ACTION_FOUND); intentFilter.addAction(BluetoothAdapter.ACTION_DISCOVERY_FINISHED); intentFilter.addAction(BluetoothDevice.ACTION_BOND_STATE_CHANGED); intentFilter.addAction(BluetoothAdapter.ACTION_CONNECTION_STATE_CHANGED); mContext.registerReceiver(receiver, intentFilter);
private final BroadcastReceiver receiver = new BroadcastReceiver() { public void onReceive(Context context, Intent intent) { String action = intent.getAction(); if (BluetoothDevice.ACTION_FOUND.equals(action)) { BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE); } else if (BluetoothAdapter.ACTION_DISCOVERY_FINISHED.equals(action)) { } else if (BluetoothDevice.ACTION_BOND_STATE_CHANGED.equals(action)) { BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE); int bondState = intent.getIntExtra(BluetoothDevice.EXTRA_BOND_STATE, BluetoothDevice.ERROR); } else if (BluetoothAdapter.ACTION_CONNECTION_STATE_CHANGED.equals(action)) { BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE); int connectState = intent.getIntExtra(BluetoothAdapter.EXTRA_CONNECTION_STATE, BluetoothAdapter.ERROR); } } };
|
2.3 查找蓝牙设备
1 2 3 4 5 6
| Set<BluetoothDevice> pairedDevices = bluetoothAdapter.getBondedDevices();
if (!bluetoothAdapter.isDiscovering()) { bluetoothAdapter.startDiscovery(); }
|
2.3 连接蓝牙设备
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| public void connectDevice(BluetoothDevice device) { if (bluetoothAdapter != null) { bluetoothAdapter.cancelDiscovery(); } if (device.getBondState() == BluetoothDevice.BOND_BONDED) { removeBondDevice(device); AppExecutors.getInstance().scheduledWork().schedule(() -> { device.createBond(); }, 1, TimeUnit.SECONDS); } else if (device.getBondState() == BluetoothDevice.BOND_NONE) { device.createBond(); } }
public static boolean isDeviceConnected(BluetoothDevice device) { boolean isConnected = false; try { isConnected = (boolean) device.getClass().getMethod("isConnected").invoke(device); } catch (Exception e) { e.printStackTrace(); } return isConnected; }
public static boolean removeBondDevice(BluetoothDevice device) { boolean state = false; try { state = (boolean) device.getClass().getMethod("removeBond").invoke(device); } catch (Exception e) { e.printStackTrace(); } return state; }
|
3.低功耗蓝牙
3.1 查找蓝牙设备
1 2 3 4 5
| AppExecutors.getInstance().scheduledWork().schedule(() -> { bluetoothAdapter.getBluetoothLeScanner().stopScan(scanCallback); }, 10, TimeUnit.SECONDS); bluetoothAdapter.getBluetoothLeScanner().startScan(scanCallback);
|
3.2 连接蓝牙设备
1 2 3 4
| bluetoothGatt = device.connectGatt(mContext, false, gattCallback, BluetoothDevice.TRANSPORT_LE)
bluetoothGatt.disconnect();
|
3.3 写入数据
1 2 3
| byte[] data; writeCharacteristic.setValue(data); bluetoothGatt.writeCharacteristic(writeCharacteristic);
|
4.效果图
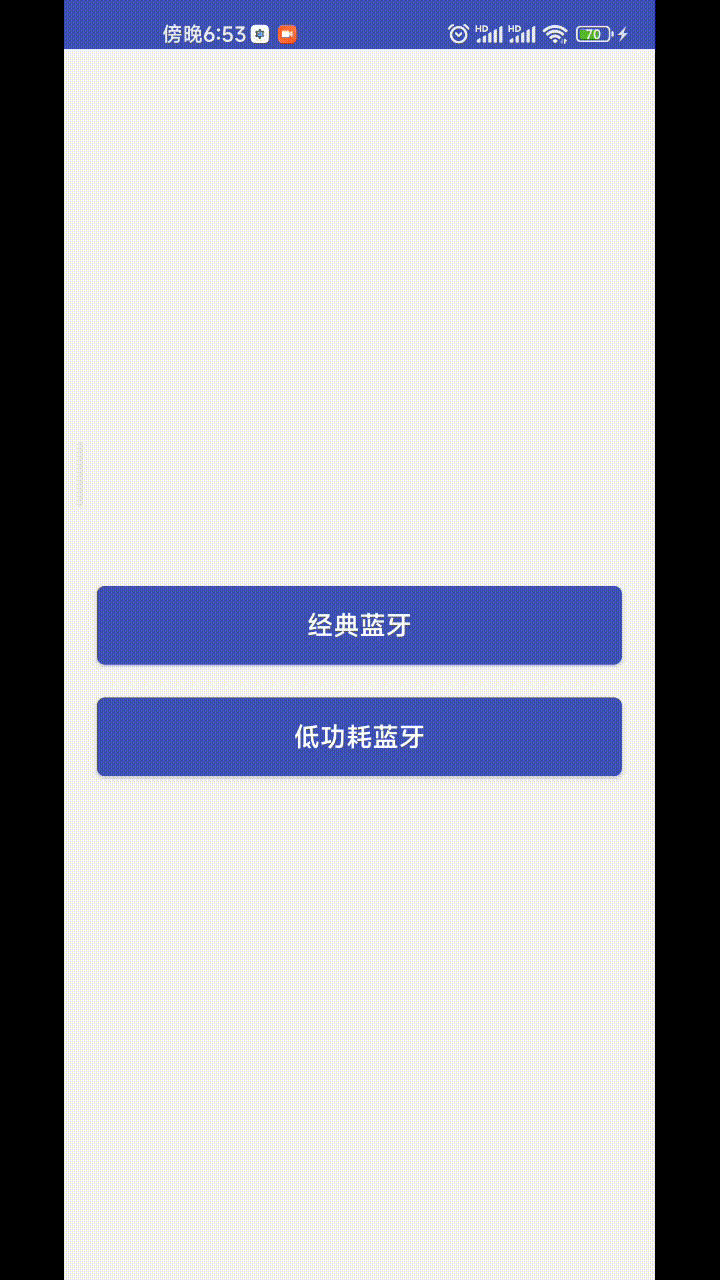
5.官方文档
通信技术-蓝牙概览
通信技术-蓝牙开发